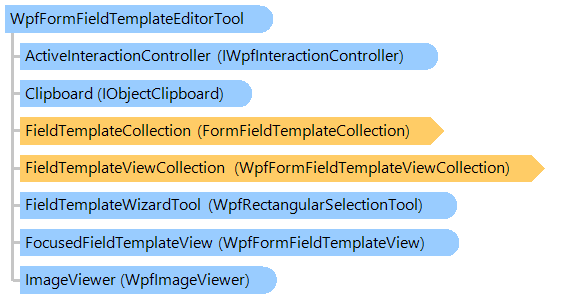
'Declaration <DesignTimeVisibleAttribute("Visible = False")> <ToolboxItemAttribute("ToolboxItemType = null", "ToolboxItemTypeName = ")> <DefaultPropertyAttribute("Content")> <ContentPropertyAttribute("Content")> <LocalizabilityAttribute(None)> <StyleTypedPropertyAttribute("Property = FocusVisualStyle", "StyleTargetType = System.Windows.Controls.Control")> <XmlLangPropertyAttribute("Name = Language")> <UsableDuringInitializationAttribute("Usable = True")> <RuntimeNamePropertyAttribute("Name = Name")> <UidPropertyAttribute()> <TypeDescriptionProviderAttribute("TypeName = MS.Internal.ComponentModel.DependencyObjectProvider")> <NameScopePropertyAttribute("Name = NameScope", "Type = System.Windows.NameScope")> Public Class WpfFormFieldTemplateEditorTool Inherits Vintasoft.Imaging.Wpf.UI.VisualTools.UserInteraction.WpfUserInteractionVisualTool
[DesignTimeVisible("Visible = False")] [ToolboxItem("ToolboxItemType = null", "ToolboxItemTypeName = ")] [DefaultProperty("Content")] [ContentProperty("Content")] [Localizability(None)] [StyleTypedProperty("Property = FocusVisualStyle", "StyleTargetType = System.Windows.Controls.Control")] [XmlLangProperty("Name = Language")] [UsableDuringInitialization("Usable = True")] [RuntimeNameProperty("Name = Name")] [UidProperty()] [TypeDescriptionProvider("TypeName = MS.Internal.ComponentModel.DependencyObjectProvider")] [NameScopeProperty("Name = NameScope", "Type = System.Windows.NameScope")] public class WpfFormFieldTemplateEditorTool : Vintasoft.Imaging.Wpf.UI.VisualTools.UserInteraction.WpfUserInteractionVisualTool
[DesignTimeVisible("Visible = False")] [ToolboxItem("ToolboxItemType = null", "ToolboxItemTypeName = ")] [DefaultProperty("Content")] [ContentProperty("Content")] [Localizability(None)] [StyleTypedProperty("Property = FocusVisualStyle", "StyleTargetType = System.Windows.Controls.Control")] [XmlLangProperty("Name = Language")] [UsableDuringInitialization("Usable = True")] [RuntimeNameProperty("Name = Name")] [UidProperty()] [TypeDescriptionProvider("TypeName = MS.Internal.ComponentModel.DependencyObjectProvider")] [NameScopeProperty("Name = NameScope", "Type = System.Windows.NameScope")] public __gc class WpfFormFieldTemplateEditorTool : public Vintasoft.Imaging.Wpf.UI.VisualTools.UserInteraction.WpfUserInteractionVisualTool*
[DesignTimeVisible("Visible = False")] [ToolboxItem("ToolboxItemType = null", "ToolboxItemTypeName = ")] [DefaultProperty("Content")] [ContentProperty("Content")] [Localizability(None)] [StyleTypedProperty("Property = FocusVisualStyle", "StyleTargetType = System.Windows.Controls.Control")] [XmlLangProperty("Name = Language")] [UsableDuringInitialization("Usable = True")] [RuntimeNameProperty("Name = Name")] [UidProperty()] [TypeDescriptionProvider("TypeName = MS.Internal.ComponentModel.DependencyObjectProvider")] [NameScopeProperty("Name = NameScope", "Type = System.Windows.NameScope")] public ref class WpfFormFieldTemplateEditorTool : public Vintasoft.Imaging.Wpf.UI.VisualTools.UserInteraction.WpfUserInteractionVisualTool^
This C#/VB.NET code shows how to create a form template and add some form field templates to it visually.
System.Object
 System.Windows.Threading.DispatcherObject
   System.Windows.DependencyObject
     System.Windows.Media.Visual
       System.Windows.UIElement
         System.Windows.FrameworkElement
           System.Windows.Controls.Control
             System.Windows.Controls.ContentControl
               Vintasoft.Imaging.Wpf.UI.VisualTools.WpfVisualTool
                 Vintasoft.Imaging.Wpf.UI.VisualTools.UserInteraction.WpfUserInteractionVisualTool
                   Vintasoft.Imaging.FormsProcessing.FormRecognition.Wpf.UI.VisualTools.WpfFormFieldTemplateEditorTool
Target Platforms: .NET9; .NET 8; .NET 7; .NET 6; .NET Framework 4.8, 4.7, 4.6, 4.5, 4.0, 3.5
WpfFormFieldTemplateEditorTool Members
Vintasoft.Imaging.FormsProcessing.FormRecognition.Wpf.UI.VisualTools Namespace