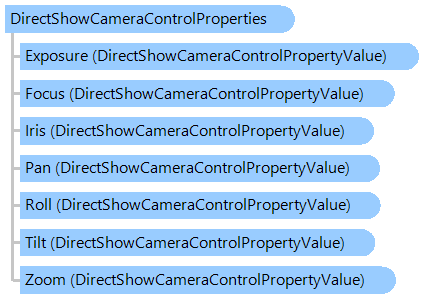
'Declaration Public Class DirectShowCameraControlProperties
public class DirectShowCameraControlProperties
public __gc class DirectShowCameraControlProperties
public ref class DirectShowCameraControlProperties
This C#/VB.NET code shows how to get and set the control properties of DirectShow camera:
''' <summary> ''' Gets and sets the camera control properties of DirectShow camera. ''' </summary> Public Shared Sub GetAndSetCameraControlProperties() ' get a list of installed cameras Dim captureDevices As System.Collections.ObjectModel.ReadOnlyCollection(Of Vintasoft.Imaging.Media.ImageCaptureDevice) = Vintasoft.Imaging.Media.ImageCaptureDeviceConfiguration.GetCaptureDevices() ' if cameras are not found If captureDevices.Count = 0 Then System.Console.WriteLine("No connected devices.") Return End If ' get the first available camera Dim camera As Vintasoft.Imaging.Media.DirectShowCamera = DirectCast(captureDevices(0), Vintasoft.Imaging.Media.DirectShowCamera) ' output camera name System.Console.WriteLine(camera.FriendlyName) Dim propertyValue As Vintasoft.Imaging.Media.DirectShowCameraControlPropertyValue Dim minValue As Integer, maxValue As Integer, [step] As Integer, defaultValue As Integer System.Console.WriteLine(" - Exposure") Try ' get supported values camera.CameraControl.GetSupportedExposureValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.CameraControl.Exposure System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.CameraControl.Exposure = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try System.Console.WriteLine(" - Focus") Try ' get supported values camera.CameraControl.GetSupportedFocusValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.CameraControl.Focus System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.CameraControl.Focus = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try System.Console.WriteLine(" - Iris") Try ' get supported values camera.CameraControl.GetSupportedIrisValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.CameraControl.Iris System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.CameraControl.Iris = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try System.Console.WriteLine(" - Pan") Try ' get supported values camera.CameraControl.GetSupportedPanValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.CameraControl.Pan System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.CameraControl.Pan = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try System.Console.WriteLine(" - Roll") Try ' get supported values camera.CameraControl.GetSupportedRollValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.CameraControl.Roll System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.CameraControl.Roll = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try System.Console.WriteLine(" - Tilt") Try ' get supported values camera.CameraControl.GetSupportedTiltValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.CameraControl.Tilt System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.CameraControl.Tilt = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try System.Console.WriteLine(" - Zoom") Try ' get supported values camera.CameraControl.GetSupportedZoomValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.CameraControl.Zoom System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.CameraControl.Zoom = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try End Sub
/// <summary> /// Gets and sets the camera control properties of DirectShow camera. /// </summary> public static void GetAndSetCameraControlProperties() { // get a list of installed cameras System.Collections.ObjectModel.ReadOnlyCollection<Vintasoft.Imaging.Media.ImageCaptureDevice> captureDevices = Vintasoft.Imaging.Media.ImageCaptureDeviceConfiguration.GetCaptureDevices(); // if cameras are not found if (captureDevices.Count == 0) { System.Console.WriteLine("No connected devices."); return; } // get the first available camera Vintasoft.Imaging.Media.DirectShowCamera camera = (Vintasoft.Imaging.Media.DirectShowCamera)captureDevices[0]; // output camera name System.Console.WriteLine(camera.FriendlyName); Vintasoft.Imaging.Media.DirectShowCameraControlPropertyValue propertyValue; int minValue, maxValue, step, defaultValue; System.Console.WriteLine(" - Exposure"); try { // get supported values camera.CameraControl.GetSupportedExposureValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.CameraControl.Exposure; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.CameraControl.Exposure = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } System.Console.WriteLine(" - Focus"); try { // get supported values camera.CameraControl.GetSupportedFocusValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.CameraControl.Focus; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.CameraControl.Focus = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } System.Console.WriteLine(" - Iris"); try { // get supported values camera.CameraControl.GetSupportedIrisValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.CameraControl.Iris; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.CameraControl.Iris = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } System.Console.WriteLine(" - Pan"); try { // get supported values camera.CameraControl.GetSupportedPanValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.CameraControl.Pan; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.CameraControl.Pan = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } System.Console.WriteLine(" - Roll"); try { // get supported values camera.CameraControl.GetSupportedRollValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.CameraControl.Roll; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.CameraControl.Roll = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } System.Console.WriteLine(" - Tilt"); try { // get supported values camera.CameraControl.GetSupportedTiltValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.CameraControl.Tilt; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.CameraControl.Tilt = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } System.Console.WriteLine(" - Zoom"); try { // get supported values camera.CameraControl.GetSupportedZoomValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.CameraControl.Zoom; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.CameraControl.Zoom = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } }
System.Object
 Vintasoft.Imaging.Media.DirectShowCameraControlProperties
Target Platforms: .NET9; .NET 8; .NET 7; .NET 6; .NET Framework 4.8, 4.7, 4.6, 4.5, 4.0, 3.5